Note
Go to the end to download the full example code or to run this example in your browser via Binder
Basic Atlas plotting¶
Plot the regions of a reference atlas (Harvard-Oxford and Juelich atlases).
Retrieving the atlas data¶
from nilearn import datasets
dataset_ho = datasets.fetch_atlas_harvard_oxford("cort-maxprob-thr25-2mm")
dataset_ju = datasets.fetch_atlas_juelich("maxprob-thr0-1mm")
atlas_ho_filename = dataset_ho.filename
atlas_ju_filename = dataset_ju.filename
print(f"Atlas ROIs are located at: {atlas_ho_filename}")
print(f"Atlas ROIs are located at: {atlas_ju_filename}")
Atlas ROIs are located at: /home/runner/work/nilearn/nilearn/nilearn_data/fsl/data/atlases/HarvardOxford/HarvardOxford-cort-maxprob-thr25-2mm.nii.gz
Atlas ROIs are located at: /home/runner/work/nilearn/nilearn/nilearn_data/fsl/data/atlases/Juelich/Juelich-maxprob-thr0-1mm.nii.gz
Visualizing the Harvard-Oxford atlas¶
from nilearn import plotting
plotting.plot_roi(atlas_ho_filename, title="Harvard Oxford atlas")
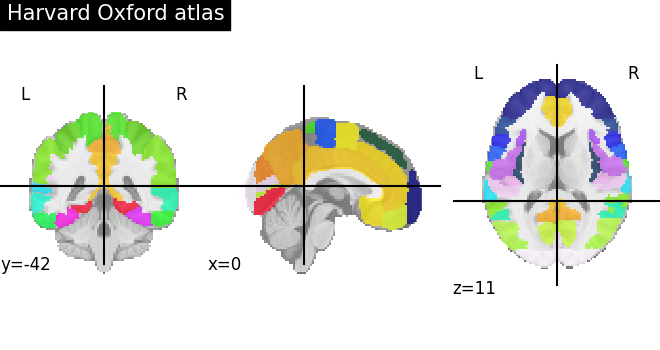
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f853db0da90>
Visualizing the Juelich atlas¶
plotting.plot_roi(atlas_ju_filename, title="Juelich atlas")
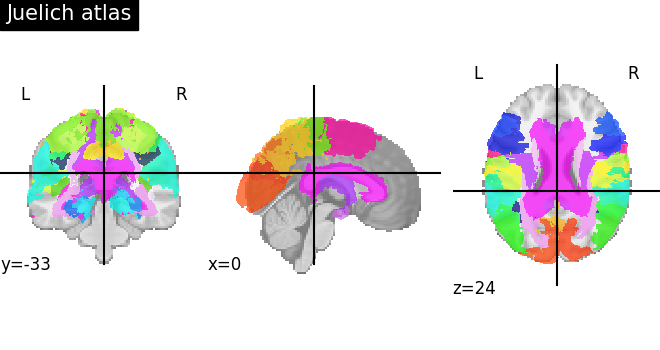
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7f852dbfdf10>
Visualizing the Harvard-Oxford atlas with contours¶
plotting.plot_roi(
atlas_ho_filename,
view_type="contours",
title="Harvard Oxford atlas in contours",
)
plotting.show()
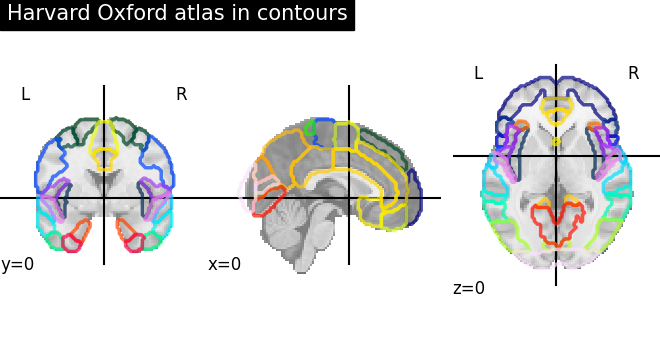
/opt/hostedtoolcache/Python/3.12.3/x64/lib/python3.12/site-packages/nilearn/plotting/img_plotting.py:817: UserWarning: Data array used to create a new image contains 64-bit ints. This is likely due to creating the array with numpy and passing `int` as the `dtype`. Many tools such as FSL and SPM cannot deal with int64 in Nifti images, so for compatibility the data has been converted to int32.
img = new_img_like(roi_img, data, affine=roi_img.affine)
Visualizing the Juelich atlas with contours¶
plotting.plot_roi(
atlas_ju_filename, view_type="contours", title="Juelich atlas in contours"
)
plotting.show()
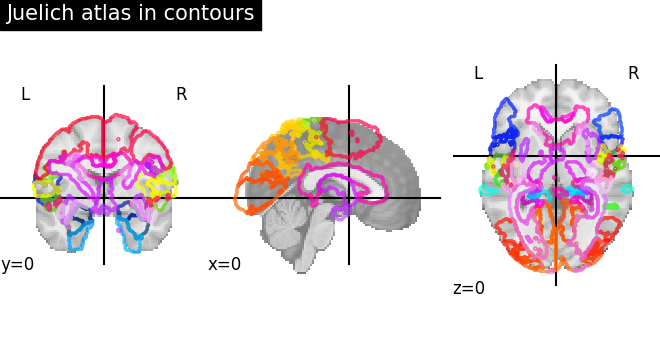
Total running time of the script: (1 minutes 11.081 seconds)
Estimated memory usage: 439 MB