Note
This page is a reference documentation. It only explains the class signature, and not how to use it. Please refer to the user guide for the big picture.
nilearn.maskers.NiftiSpheresMasker#
- class nilearn.maskers.NiftiSpheresMasker(seeds, radius=None, mask_img=None, allow_overlap=False, smoothing_fwhm=None, standardize=False, standardize_confounds=True, high_variance_confounds=False, detrend=False, low_pass=None, high_pass=None, t_r=None, dtype=None, memory=Memory(location=None), memory_level=1, verbose=0, reports=True, **kwargs)[source]#
Class for masking of Niimg-like objects using seeds.
NiftiSpheresMasker is useful when data from given seeds should be extracted.
Use case: summarize brain signals from seeds that were obtained from prior knowledge.
- Parameters:
- seeds
list
of triplet of coordinates in native space Seed definitions. List of coordinates of the seeds in the same space as the images (typically MNI or TAL).
- radius
float
, default=None Indicates, in millimeters, the radius for the sphere around the seed. By default signal is extracted on a single voxel.
- mask_imgNiimg-like object, default=None
See Input and output: neuroimaging data representation. Mask to apply to regions before extracting signals.
- allow_overlap
bool
, default=False If False, an error is raised if the maps overlaps (ie at least two maps have a non-zero value for the same voxel).
- smoothing_fwhm
float
, optional. If smoothing_fwhm is not None, it gives the full-width at half maximum in millimeters of the spatial smoothing to apply to the signal.
- standardize{‘zscore_sample’, ‘zscore’, ‘psc’, True, False}, default=False
Strategy to standardize the signal:
‘zscore_sample’: The signal is z-scored. Timeseries are shifted to zero mean and scaled to unit variance. Uses sample std.
‘zscore’: The signal is z-scored. Timeseries are shifted to zero mean and scaled to unit variance. Uses population std by calling default
numpy.std
with N -ddof=0
.‘psc’: Timeseries are shifted to zero mean value and scaled to percent signal change (as compared to original mean signal).
True: The signal is z-scored (same as option zscore). Timeseries are shifted to zero mean and scaled to unit variance.
False: Do not standardize the data.
- standardize_confounds
bool
, default=True If set to True, the confounds are z-scored: their mean is put to 0 and their variance to 1 in the time dimension.
- high_variance_confounds
bool
, default=False If True, high variance confounds are computed on provided image with
nilearn.image.high_variance_confounds
and default parameters and regressed out.- detrend
bool
, optional Whether to detrend signals or not.
- low_pass
float
or None, default=None Low cutoff frequency in Hertz. If specified, signals above this frequency will be filtered out. If None, no low-pass filtering will be performed.
- high_pass
float
, default=None High cutoff frequency in Hertz. If specified, signals below this frequency will be filtered out.
- t_r
float
or None, default=None Repetition time, in seconds (sampling period). Set to None if not provided.
- dtype{dtype, “auto”}, optional
Data type toward which the data should be converted. If “auto”, the data will be converted to int32 if dtype is discrete and float32 if it is continuous.
- memoryinstance of
joblib.Memory
,str
, orpathlib.Path
Used to cache the masking process. By default, no caching is done. If a
str
is given, it is the path to the caching directory.- memory_level
int
, default=1 Rough estimator of the amount of memory used by caching. Higher value means more memory for caching. Zero means no caching.
- verbose
int
, default=0 Verbosity level (0 means no message).
- kwargsdict
Keyword arguments to be passed to functions called within the masker. Kwargs prefixed with ‘clean__’ will be passed to
clean
. Withinclean
, kwargs prefixed with ‘butterworth__’ will be passed to the Butterworth filter (i.e., clean__butterworth__).
- seeds
See also
- Attributes:
- __init__(seeds, radius=None, mask_img=None, allow_overlap=False, smoothing_fwhm=None, standardize=False, standardize_confounds=True, high_variance_confounds=False, detrend=False, low_pass=None, high_pass=None, t_r=None, dtype=None, memory=Memory(location=None), memory_level=1, verbose=0, reports=True, **kwargs)[source]#
- generate_report(displayed_spheres='all')[source]#
Generate an HTML report for current
NiftiSpheresMasker
object.Note
This functionality requires to have
Matplotlib
installed.- Parameters:
- displayed_spheres
int
, orlist
, orndarray
, or “all”, default=”all” Indicates which spheres will be displayed in the HTML report.
If “all”: All spheres will be displayed in the report.
masker.generate_report("all")
Warning
If there are too many spheres, this might be time and memory consuming, and will result in very heavy reports.
If a
list
orndarray
: This indicates the indices of the spheres to be displayed in the report. For example, the following code will generate a report with spheres 6, 3, and 12, displayed in this specific order:
masker.generate_report([6, 3, 12])
If an
int
: This will only display the first n spheres, n being the value of the parameter. By default, the report will only contain the first 10 spheres. Example to display the first 16 spheres:
masker.generate_report(16)
- displayed_spheres
- Returns:
- reportnilearn.reporting.html_report.HTMLReport
HTML report for the masker.
- fit(X=None, y=None)[source]#
Prepare signal extraction from regions.
All parameters are unused; they are for scikit-learn compatibility.
- fit_transform(imgs, confounds=None, sample_mask=None)[source]#
Prepare and perform signal extraction.
- Parameters:
- imgs3D/4D Niimg-like object
See Input and output: neuroimaging data representation. Images to process. If a 3D niimg is provided, a singleton dimension will be added to the output to represent the single scan in the niimg.
- confoundsCSV file or array-like or
pandas.DataFrame
, optional This parameter is passed to signal.clean. Please see the related documentation for details. shape: (number of scans, number of confounds)
- sample_maskAny type compatible with numpy-array indexing, optional
Masks the niimgs along time/fourth dimension to perform scrubbing (remove volumes with high motion) and/or non-steady-state volumes. This parameter is passed to signal.clean. shape: (number of scans - number of volumes removed, )
New in version 0.8.0.
- Returns:
- region_signals2D
numpy.ndarray
Signal for each sphere. shape: (number of scans, number of spheres)
- region_signals2D
- transform_single_imgs(imgs, confounds=None, sample_mask=None)[source]#
Extract signals from a single 4D niimg.
- Parameters:
- imgs3D/4D Niimg-like object
See Input and output: neuroimaging data representation. Images to process. If a 3D niimg is provided, a singleton dimension will be added to the output to represent the single scan in the niimg.
- confoundsCSV file or array-like or
pandas.DataFrame
, optional This parameter is passed to signal.clean. Please see the related documentation for details. shape: (number of scans, number of confounds)
- sample_maskAny type compatible with numpy-array indexing, optional
Masks the niimgs along time/fourth dimension to perform scrubbing (remove volumes with high motion) and/or non-steady-state volumes. This parameter is passed to signal.clean. shape: (number of scans - number of volumes removed, )
New in version 0.8.0.
- Returns:
- region_signals2D
numpy.ndarray
Signal for each sphere. shape: (number of scans, number of spheres)
- region_signals2D
- Warns:
- DeprecationWarning
If a 3D niimg input is provided, the current behavior (adding a singleton dimension to produce a 2D array) is deprecated. Starting in version 0.12, a 1D array will be returned for 3D inputs.
- inverse_transform(region_signals)[source]#
Compute voxel signals from spheres signals.
Any mask given at initialization is taken into account. Throws an error if
mask_img==None
- Parameters:
- region_signals1D/2D
numpy.ndarray
Signal for each region. If a 1D array is provided, then the shape should be (number of elements,), and a 3D img will be returned. If a 2D array is provided, then the shape should be (number of scans, number of elements), and a 4D img will be returned.
- region_signals1D/2D
- Returns:
- voxel_signals
nibabel.nifti1.Nifti1Image
Signal for each sphere. shape: (mask_img, number of scans).
- voxel_signals
- get_metadata_routing()#
Get metadata routing of this object.
Please check User Guide on how the routing mechanism works.
- Returns:
- routingMetadataRequest
A
MetadataRequest
encapsulating routing information.
- get_params(deep=True)#
Get parameters for this estimator.
- Parameters:
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns:
- paramsdict
Parameter names mapped to their values.
- set_inverse_transform_request(*, region_signals='$UNCHANGED$')#
Request metadata passed to the
inverse_transform
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed toinverse_transform
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it toinverse_transform
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- region_signalsstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
region_signals
parameter ininverse_transform
.
- Returns:
- selfobject
The updated object.
- set_output(*, transform=None)#
Set output container.
See Introducing the set_output API for an example on how to use the API.
- Parameters:
- transform{“default”, “pandas”}, default=None
Configure output of transform and fit_transform.
“default”: Default output format of a transformer
“pandas”: DataFrame output
“polars”: Polars output
None: Transform configuration is unchanged
New in version 1.4: “polars” option was added.
- Returns:
- selfestimator instance
Estimator instance.
- set_params(**params)#
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters:
- **paramsdict
Estimator parameters.
- Returns:
- selfestimator instance
Estimator instance.
- set_transform_request(*, confounds='$UNCHANGED$', imgs='$UNCHANGED$', sample_mask='$UNCHANGED$')#
Request metadata passed to the
transform
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed totransform
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it totransform
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- confoundsstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
confounds
parameter intransform
.- imgsstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
imgs
parameter intransform
.- sample_maskstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
sample_mask
parameter intransform
.
- Returns:
- selfobject
The updated object.
- transform(imgs, confounds=None, sample_mask=None)[source]#
Apply mask, spatial and temporal preprocessing.
- Parameters:
- imgs3D/4D Niimg-like object
See Input and output: neuroimaging data representation. Images to process. If a 3D niimg is provided, a singleton dimension will be added to the output to represent the single scan in the niimg.
- confoundsCSV file or array-like, optional
This parameter is passed to signal.clean. Please see the related documentation for details. shape: (number of scans, number of confounds)
- sample_maskAny type compatible with numpy-array indexing, optional
shape: (number of scans - number of volumes removed, ) Masks the niimgs along time/fourth dimension to perform scrubbing (remove volumes with high motion) and/or non-steady-state volumes. This parameter is passed to signal.clean.
New in version 0.8.0.
- Returns:
- region_signals2D numpy.ndarray
Signal for each element. shape: (number of scans, number of elements)
- Warns:
- DeprecationWarning
If a 3D niimg input is provided, the current behavior (adding a singleton dimension to produce a 2D array) is deprecated. Starting in version 0.12, a 1D array will be returned for 3D inputs.
Examples using nilearn.maskers.NiftiSpheresMasker
#
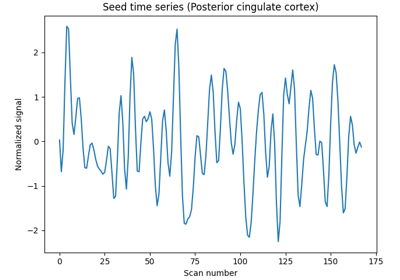
Producing single subject maps of seed-to-voxel correlation
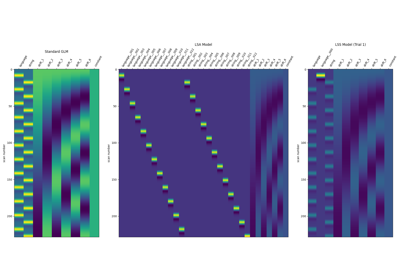
Beta-Series Modeling for Task-Based Functional Connectivity and Decoding