Note
Go to the end to download the full example code or to run this example in your browser via Binder
Extracting signals from brain regions using the NiftiLabelsMasker#
This simple example shows how to extract signals from functional
fMRI data and brain regions defined through an atlas.
More precisely, this example shows how to use the
NiftiLabelsMasker
object to perform this
operation in just a few lines of code.
Note
If you are using Nilearn with a version older than 0.9.0
,
then you should either upgrade your version or import maskers
from the input_data
module instead of the maskers
module.
That is, you should manually replace in the following example all occurrences of:
from nilearn.maskers import NiftiMasker
with:
from nilearn.input_data import NiftiMasker
try:
import matplotlib.pyplot as plt
except ImportError:
raise RuntimeError("This script needs the matplotlib library")
Retrieve the brain development functional dataset
We start by fetching the brain development functional dataset and we restrict the example to one subject only.
from nilearn import datasets
dataset = datasets.fetch_development_fmri(n_subjects=1)
func_filename = dataset.func[0]
# print basic information on the dataset
print(f"First functional nifti image (4D) is at: {func_filename}")
First functional nifti image (4D) is at: /home/himanshu/nilearn_data/development_fmri/development_fmri/sub-pixar123_task-pixar_space-MNI152NLin2009cAsym_desc-preproc_bold.nii.gz
Load an atlas
We then load the Harvard-Oxford atlas to define the brain regions
atlas = datasets.fetch_atlas_harvard_oxford("cort-maxprob-thr25-2mm")
# The first label correspond to the background
print(f"The atlas contains {len(atlas.labels) - 1} non-overlapping regions")
The atlas contains 48 non-overlapping regions
Instantiate the mask and visualize atlas
from nilearn.maskers import NiftiLabelsMasker
# Instantiate the masker with label image and label values
masker = NiftiLabelsMasker(
atlas.maps,
labels=atlas.labels,
standardize="zscore_sample",
)
# Visualize the atlas
# Note that we need to call fit prior to generating the mask
masker.fit()
# At this point, no functional image has been provided to the masker.
# We can still generate a report which can be displayed in a Jupyter
# Notebook, opened in a browser using the .open_in_browser() method,
# or saved to a file using the .save_as_html(output_filepath) method.
report = masker.generate_report()
report
/home/himanshu/Desktop/nilearn_work/nilearn/examples/06_manipulating_images/plot_nifti_labels_simple.py:63: UserWarning: No image provided to fit in NiftiLabelsMasker. Plotting ROIs of label image on the MNI152Template for reporting.
report = masker.generate_report()
NiftiLabelsMasker Class for extracting data from Niimg-like objects using labels of non-overlapping brain regions. NiftiLabelsMasker is useful when data from non-overlapping volumes should be extracted (contrarily to :class:`nilearn.maskers.NiftiMapsMasker`). Use case: summarize brain signals from clusters that were obtained by prior K-means or Ward clustering. For more details on the definitions of labels in Nilearn, see the :ref:`region` section.
No image provided to fit in NiftiLabelsMasker. Plotting ROIs of label image on the MNI152Template for reporting.
This reports shows the regions defined by the labels of the mask.
The masker has 48 different non-overlapping regions.
Regions summary
label value | region name | size (in mm^3) | relative size (in %) |
---|---|---|---|
1 | Frontal Pole | 123176 | 11.75 |
2 | Insular Cortex | 18728 | 1.79 |
3 | Superior Frontal Gyrus | 40640 | 3.88 |
4 | Middle Frontal Gyrus | 42528 | 4.06 |
5 | Inferior Frontal Gyrus, pars triangularis | 8824 | 0.84 |
6 | Inferior Frontal Gyrus, pars opercularis | 11072 | 1.06 |
7 | Precentral Gyrus | 68584 | 6.54 |
8 | Temporal Pole | 37688 | 3.59 |
9 | Superior Temporal Gyrus, anterior division | 4168 | 0.4 |
10 | Superior Temporal Gyrus, posterior division | 14640 | 1.4 |
11 | Middle Temporal Gyrus, anterior division | 6784 | 0.65 |
12 | Middle Temporal Gyrus, posterior division | 20200 | 1.93 |
13 | Middle Temporal Gyrus, temporooccipital part | 16032 | 1.53 |
14 | Inferior Temporal Gyrus, anterior division | 5176 | 0.49 |
15 | Inferior Temporal Gyrus, posterior division | 15536 | 1.48 |
16 | Inferior Temporal Gyrus, temporooccipital part | 11760 | 1.12 |
17 | Postcentral Gyrus | 55160 | 5.26 |
18 | Superior Parietal Lobule | 23264 | 2.22 |
19 | Supramarginal Gyrus, anterior division | 13936 | 1.33 |
20 | Supramarginal Gyrus, posterior division | 18072 | 1.72 |
21 | Angular Gyrus | 19272 | 1.84 |
22 | Lateral Occipital Cortex, superior division | 78232 | 7.46 |
23 | Lateral Occipital Cortex, inferior division | 32712 | 3.12 |
24 | Intracalcarine Cortex | 11208 | 1.07 |
25 | Frontal Medial Cortex | 7808 | 0.74 |
26 | Juxtapositional Lobule Cortex (formerly Supplementary Motor Cortex) | 11872 | 1.13 |
27 | Subcallosal Cortex | 9136 | 0.87 |
28 | Paracingulate Gyrus | 23552 | 2.25 |
29 | Cingulate Gyrus, anterior division | 20736 | 1.98 |
30 | Cingulate Gyrus, posterior division | 19296 | 1.84 |
31 | Precuneous Cortex | 44984 | 4.29 |
32 | Cuneal Cortex | 9816 | 0.94 |
33 | Frontal Orbital Cortex | 25184 | 2.4 |
34 | Parahippocampal Gyrus, anterior division | 9984 | 0.95 |
35 | Parahippocampal Gyrus, posterior division | 5680 | 0.54 |
36 | Lingual Gyrus | 27048 | 2.58 |
37 | Temporal Fusiform Cortex, anterior division | 4880 | 0.47 |
38 | Temporal Fusiform Cortex, posterior division | 12752 | 1.22 |
39 | Temporal Occipital Fusiform Cortex | 11752 | 1.12 |
40 | Occipital Fusiform Gyrus | 14448 | 1.38 |
41 | Frontal Opercular Cortex | 5496 | 0.52 |
42 | Central Opercular Cortex | 15088 | 1.44 |
43 | Parietal Opercular Cortex | 8952 | 0.85 |
44 | Planum Polare | 5992 | 0.57 |
45 | Heschl's Gyrus (includes H1 and H2) | 4832 | 0.46 |
46 | Planum Temporale | 7616 | 0.73 |
47 | Supracalcarine Cortex | 2088 | 0.2 |
48 | Occipital Pole | 42208 | 4.03 |
Parameters
Parameter | Value |
---|---|
background_label | 0 |
detrend | False |
dtype | None |
high_pass | None |
high_variance_confounds | False |
keep_masked_labels | True |
labels | ['Background', 'Frontal Pole', 'Insular Cortex', 'Superior Frontal Gyrus', 'Middle Frontal Gyrus', 'Inferior Frontal Gyrus, pars triangularis', 'Inferior Frontal Gyrus, pars opercularis', 'Precentral Gyrus', 'Temporal Pole', 'Superior Temporal Gyrus, anterior division', 'Superior Temporal Gyrus, posterior division', 'Middle Temporal Gyrus, anterior division', 'Middle Temporal Gyrus, posterior division', 'Middle Temporal Gyrus, temporooccipital part', 'Inferior Temporal Gyrus, anterior division', 'Inferior Temporal Gyrus, posterior division', 'Inferior Temporal Gyrus, temporooccipital part', 'Postcentral Gyrus', 'Superior Parietal Lobule', 'Supramarginal Gyrus, anterior division', 'Supramarginal Gyrus, posterior division', 'Angular Gyrus', 'Lateral Occipital Cortex, superior division', 'Lateral Occipital Cortex, inferior division', 'Intracalcarine Cortex', 'Frontal Medial Cortex', 'Juxtapositional Lobule Cortex (formerly Supplementary Motor Cortex)', 'Subcallosal Cortex', 'Paracingulate Gyrus', 'Cingulate Gyrus, anterior division', 'Cingulate Gyrus, posterior division', 'Precuneous Cortex', 'Cuneal Cortex', 'Frontal Orbital Cortex', 'Parahippocampal Gyrus, anterior division', 'Parahippocampal Gyrus, posterior division', 'Lingual Gyrus', 'Temporal Fusiform Cortex, anterior division', 'Temporal Fusiform Cortex, posterior division', 'Temporal Occipital Fusiform Cortex', 'Occipital Fusiform Gyrus', 'Frontal Opercular Cortex', 'Central Opercular Cortex', 'Parietal Opercular Cortex', 'Planum Polare', "Heschl's Gyrus (includes H1 and H2)", 'Planum Temporale', 'Supracalcarine Cortex', 'Occipital Pole'] |
labels_img | <class 'nibabel.nifti1.Nifti1Image'> data shape (91, 109, 91) affine: [[ 2. 0. 0. -90.] [ 0. 2. 0. -126.] [ 0. 0. 2. -72.] [ 0. 0. 0. 1.]] metadata: <class 'nibabel.nifti1.Nifti1Header'> object, endian='<' sizeof_hdr : 348 data_type : b'' db_name : b'' extents : 0 session_error : 0 regular : b'' dim_info : 0 dim : [ 3 91 109 91 1 1 1 1] intent_p1 : 0.0 intent_p2 : 0.0 intent_p3 : 0.0 intent_code : none datatype : uint8 bitpix : 8 slice_start : 0 pixdim : [1. 2. 2. 2. 1. 1. 1. 1.] vox_offset : 0.0 scl_slope : nan scl_inter : nan slice_end : 0 slice_code : unknown xyzt_units : 0 cal_max : 0.0 cal_min : 0.0 slice_duration : 0.0 toffset : 0.0 glmax : 0 glmin : 0 descrip : b'' aux_file : b'' qform_code : unknown sform_code : aligned quatern_b : 0.0 quatern_c : 0.0 quatern_d : 0.0 qoffset_x : -90.0 qoffset_y : -126.0 qoffset_z : -72.0 srow_x : [ 2. 0. 0. -90.] srow_y : [ 0. 2. 0. -126.] srow_z : [ 0. 0. 2. -72.] intent_name : b'' magic : b'n+1' |
low_pass | None |
mask_img | None |
memory | Memory(location=None) |
memory_level | 1 |
reports | True |
resampling_target | data |
smoothing_fwhm | None |
standardize | zscore_sample |
standardize_confounds | True |
strategy | mean |
t_r | None |
verbose | 0 |
This report was generated based on information provided at instantiation and fit time. Note that the masker can potentially perform resampling at transform time.
Fitting the mask and generating a report
masker.fit(func_filename)
# We can again generate a report, but this time, the provided functional
# image is displayed with the ROI of the atlas.
# The report also contains a summary table giving the region sizes in mm3
report = masker.generate_report()
report
NiftiLabelsMasker Class for extracting data from Niimg-like objects using labels of non-overlapping brain regions. NiftiLabelsMasker is useful when data from non-overlapping volumes should be extracted (contrarily to :class:`nilearn.maskers.NiftiMapsMasker`). Use case: summarize brain signals from clusters that were obtained by prior K-means or Ward clustering. For more details on the definitions of labels in Nilearn, see the :ref:`region` section.
This reports shows the regions defined by the labels of the mask.
The masker has 48 different non-overlapping regions.
Regions summary
label value | region name | size (in mm^3) | relative size (in %) |
---|---|---|---|
1 | Frontal Pole | 123176 | 11.75 |
2 | Insular Cortex | 18728 | 1.79 |
3 | Superior Frontal Gyrus | 40640 | 3.88 |
4 | Middle Frontal Gyrus | 42528 | 4.06 |
5 | Inferior Frontal Gyrus, pars triangularis | 8824 | 0.84 |
6 | Inferior Frontal Gyrus, pars opercularis | 11072 | 1.06 |
7 | Precentral Gyrus | 68584 | 6.54 |
8 | Temporal Pole | 37688 | 3.59 |
9 | Superior Temporal Gyrus, anterior division | 4168 | 0.4 |
10 | Superior Temporal Gyrus, posterior division | 14640 | 1.4 |
11 | Middle Temporal Gyrus, anterior division | 6784 | 0.65 |
12 | Middle Temporal Gyrus, posterior division | 20200 | 1.93 |
13 | Middle Temporal Gyrus, temporooccipital part | 16032 | 1.53 |
14 | Inferior Temporal Gyrus, anterior division | 5176 | 0.49 |
15 | Inferior Temporal Gyrus, posterior division | 15536 | 1.48 |
16 | Inferior Temporal Gyrus, temporooccipital part | 11760 | 1.12 |
17 | Postcentral Gyrus | 55160 | 5.26 |
18 | Superior Parietal Lobule | 23264 | 2.22 |
19 | Supramarginal Gyrus, anterior division | 13936 | 1.33 |
20 | Supramarginal Gyrus, posterior division | 18072 | 1.72 |
21 | Angular Gyrus | 19272 | 1.84 |
22 | Lateral Occipital Cortex, superior division | 78232 | 7.46 |
23 | Lateral Occipital Cortex, inferior division | 32712 | 3.12 |
24 | Intracalcarine Cortex | 11208 | 1.07 |
25 | Frontal Medial Cortex | 7808 | 0.74 |
26 | Juxtapositional Lobule Cortex (formerly Supplementary Motor Cortex) | 11872 | 1.13 |
27 | Subcallosal Cortex | 9136 | 0.87 |
28 | Paracingulate Gyrus | 23552 | 2.25 |
29 | Cingulate Gyrus, anterior division | 20736 | 1.98 |
30 | Cingulate Gyrus, posterior division | 19296 | 1.84 |
31 | Precuneous Cortex | 44984 | 4.29 |
32 | Cuneal Cortex | 9816 | 0.94 |
33 | Frontal Orbital Cortex | 25184 | 2.4 |
34 | Parahippocampal Gyrus, anterior division | 9984 | 0.95 |
35 | Parahippocampal Gyrus, posterior division | 5680 | 0.54 |
36 | Lingual Gyrus | 27048 | 2.58 |
37 | Temporal Fusiform Cortex, anterior division | 4880 | 0.47 |
38 | Temporal Fusiform Cortex, posterior division | 12752 | 1.22 |
39 | Temporal Occipital Fusiform Cortex | 11752 | 1.12 |
40 | Occipital Fusiform Gyrus | 14448 | 1.38 |
41 | Frontal Opercular Cortex | 5496 | 0.52 |
42 | Central Opercular Cortex | 15088 | 1.44 |
43 | Parietal Opercular Cortex | 8952 | 0.85 |
44 | Planum Polare | 5992 | 0.57 |
45 | Heschl's Gyrus (includes H1 and H2) | 4832 | 0.46 |
46 | Planum Temporale | 7616 | 0.73 |
47 | Supracalcarine Cortex | 2088 | 0.2 |
48 | Occipital Pole | 42208 | 4.03 |
Parameters
Parameter | Value |
---|---|
background_label | 0 |
detrend | False |
dtype | None |
high_pass | None |
high_variance_confounds | False |
keep_masked_labels | True |
labels | ['Background', 'Frontal Pole', 'Insular Cortex', 'Superior Frontal Gyrus', 'Middle Frontal Gyrus', 'Inferior Frontal Gyrus, pars triangularis', 'Inferior Frontal Gyrus, pars opercularis', 'Precentral Gyrus', 'Temporal Pole', 'Superior Temporal Gyrus, anterior division', 'Superior Temporal Gyrus, posterior division', 'Middle Temporal Gyrus, anterior division', 'Middle Temporal Gyrus, posterior division', 'Middle Temporal Gyrus, temporooccipital part', 'Inferior Temporal Gyrus, anterior division', 'Inferior Temporal Gyrus, posterior division', 'Inferior Temporal Gyrus, temporooccipital part', 'Postcentral Gyrus', 'Superior Parietal Lobule', 'Supramarginal Gyrus, anterior division', 'Supramarginal Gyrus, posterior division', 'Angular Gyrus', 'Lateral Occipital Cortex, superior division', 'Lateral Occipital Cortex, inferior division', 'Intracalcarine Cortex', 'Frontal Medial Cortex', 'Juxtapositional Lobule Cortex (formerly Supplementary Motor Cortex)', 'Subcallosal Cortex', 'Paracingulate Gyrus', 'Cingulate Gyrus, anterior division', 'Cingulate Gyrus, posterior division', 'Precuneous Cortex', 'Cuneal Cortex', 'Frontal Orbital Cortex', 'Parahippocampal Gyrus, anterior division', 'Parahippocampal Gyrus, posterior division', 'Lingual Gyrus', 'Temporal Fusiform Cortex, anterior division', 'Temporal Fusiform Cortex, posterior division', 'Temporal Occipital Fusiform Cortex', 'Occipital Fusiform Gyrus', 'Frontal Opercular Cortex', 'Central Opercular Cortex', 'Parietal Opercular Cortex', 'Planum Polare', "Heschl's Gyrus (includes H1 and H2)", 'Planum Temporale', 'Supracalcarine Cortex', 'Occipital Pole'] |
labels_img | <class 'nibabel.nifti1.Nifti1Image'> data shape (91, 109, 91) affine: [[ 2. 0. 0. -90.] [ 0. 2. 0. -126.] [ 0. 0. 2. -72.] [ 0. 0. 0. 1.]] metadata: <class 'nibabel.nifti1.Nifti1Header'> object, endian='<' sizeof_hdr : 348 data_type : b'' db_name : b'' extents : 0 session_error : 0 regular : b'' dim_info : 0 dim : [ 3 91 109 91 1 1 1 1] intent_p1 : 0.0 intent_p2 : 0.0 intent_p3 : 0.0 intent_code : none datatype : uint8 bitpix : 8 slice_start : 0 pixdim : [1. 2. 2. 2. 1. 1. 1. 1.] vox_offset : 0.0 scl_slope : nan scl_inter : nan slice_end : 0 slice_code : unknown xyzt_units : 0 cal_max : 0.0 cal_min : 0.0 slice_duration : 0.0 toffset : 0.0 glmax : 0 glmin : 0 descrip : b'' aux_file : b'' qform_code : unknown sform_code : aligned quatern_b : 0.0 quatern_c : 0.0 quatern_d : 0.0 qoffset_x : -90.0 qoffset_y : -126.0 qoffset_z : -72.0 srow_x : [ 2. 0. 0. -90.] srow_y : [ 0. 2. 0. -126.] srow_z : [ 0. 0. 2. -72.] intent_name : b'' magic : b'n+1' |
low_pass | None |
mask_img | None |
memory | Memory(location=None) |
memory_level | 1 |
reports | True |
resampling_target | data |
smoothing_fwhm | None |
standardize | zscore_sample |
standardize_confounds | True |
strategy | mean |
t_r | None |
verbose | 0 |
This report was generated based on information provided at instantiation and fit time. Note that the masker can potentially perform resampling at transform time.
Process the data with the NiftiLablesMasker
In order to extract the signals, we need to call transform on the functional data
signals = masker.transform(func_filename)
# signals is a 2D matrix, (n_time_points x n_regions)
signals.shape
(168, 48)
Plot the signals
fig = plt.figure(figsize=(15, 5))
ax = fig.add_subplot(111)
for label_idx in range(3):
ax.plot(
signals[:, label_idx], linewidth=2, label=atlas.labels[label_idx + 1]
) # 0 is background
ax.legend(loc=2)
ax.set_title("Signals for first 3 regions")
plt.show()
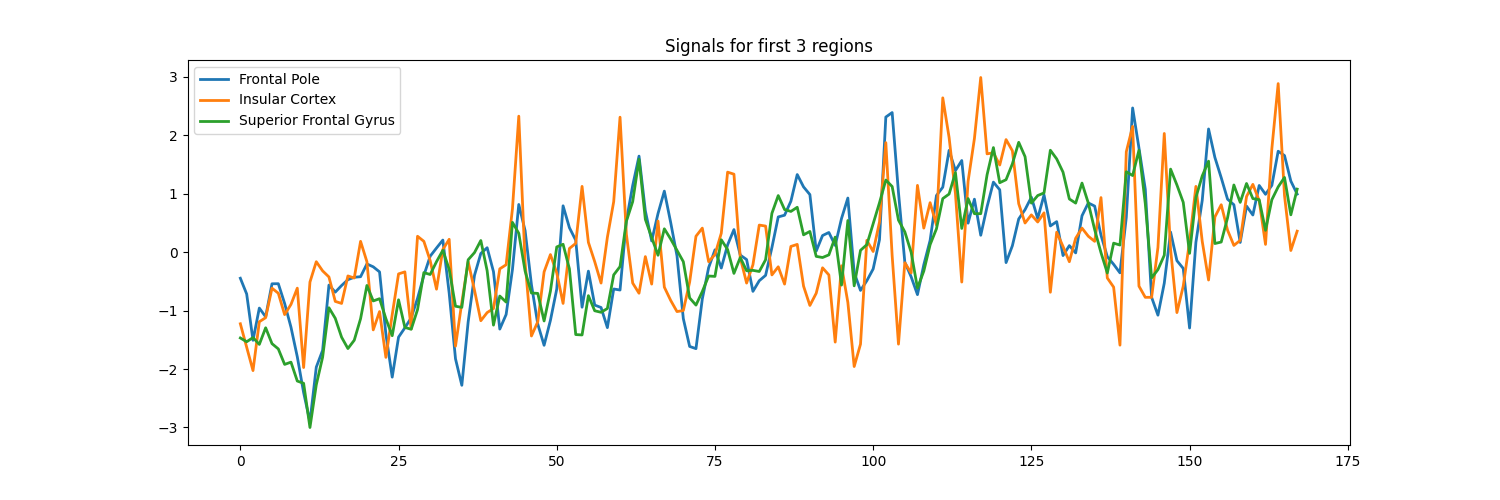
Total running time of the script: (0 minutes 7.345 seconds)
Estimated memory usage: 640 MB