Note
Go to the end to download the full example code. or to run this example in your browser via Binder
3D and 4D niimgs: handling and visualizing¶
Here we discover how to work with 3D and 4D niimgs.
Downloading tutorial datasets from Internet¶
Nilearn comes with functions that download public data from Internet
Let’s first check where the data is downloaded on our disk:
from nilearn import datasets
print(f"Datasets are stored in: {datasets.get_data_dirs()!r}")
Datasets are stored in: ['/home/runner/nilearn_data']
Let’s now retrieve a motor contrast from a Neurovault repository
[get_dataset_dir] Dataset created in /home/runner/nilearn_data/neurovault
['/home/runner/nilearn_data/neurovault/collection_658/image_10426.nii.gz']
motor_images is a list of filenames. We need to take the first one
Visualizing a 3D file¶
The file contains a 3D volume, we can easily visualize it as a statistical map:
from nilearn.plotting import plot_stat_map, show
plot_stat_map(tmap_filename)
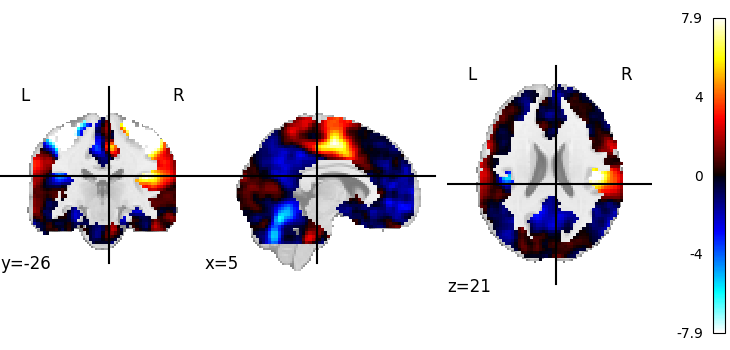
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7ff0163902b0>
Visualizing works better with a threshold
plot_stat_map(tmap_filename, threshold=3)
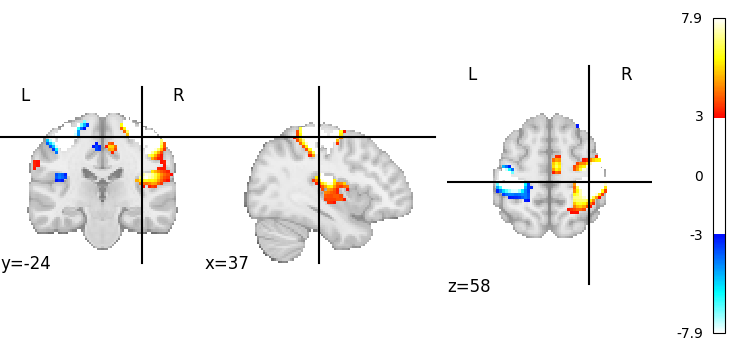
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7ff01621fa30>
Visualizing one volume in a 4D file¶
We can download resting-state networks from the Smith 2009 study on correspondence between rest and task
rsn = datasets.fetch_atlas_smith_2009(resting=True, dimension=10)["maps"]
rsn
[get_dataset_dir] Dataset created in /home/runner/nilearn_data/smith_2009
[fetch_single_file] Downloading data from
https://www.fmrib.ox.ac.uk/datasets/brainmap+rsns/PNAS_Smith09_rsn10.nii.gz ...
[fetch_single_file] ...done. (3 seconds, 0 min)
'/home/runner/nilearn_data/smith_2009/PNAS_Smith09_rsn10.nii.gz'
It is a 4D nifti file. We load it into the memory to print its shape.
from nilearn import image
print(image.load_img(rsn).shape)
(91, 109, 91, 10)
We can retrieve the first volume (note that Python indexing starts at 0):
first_rsn = image.index_img(rsn, 0)
print(first_rsn.shape)
(91, 109, 91)
first_rsn is a 3D image.
We can then plot it
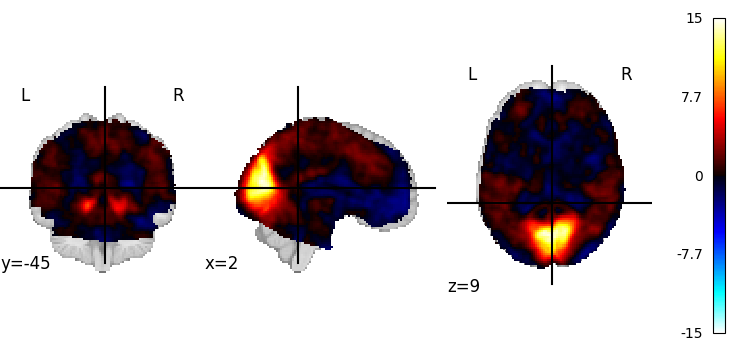
<nilearn.plotting.displays._slicers.OrthoSlicer object at 0x7ff0161f8760>
Looping on all volumes in a 4D file¶
If we want to plot all the volumes in this 4D file, we can use iter_img to loop on them.
Then we give a few arguments to plot_stat_map in order to have a more compact display.
for img in image.iter_img(rsn):
# img is now an in-memory 3D img
plot_stat_map(
img, threshold=3, display_mode="z", cut_coords=1, colorbar=False
)
/home/runner/work/nilearn/nilearn/.tox/doc/lib/python3.9/site-packages/nilearn/plotting/displays/_slicers.py:1509: MatplotlibDeprecationWarning: Adding an axes using the same arguments as a previous axes currently reuses the earlier instance. In a future version, a new instance will always be created and returned. Meanwhile, this warning can be suppressed, and the future behavior ensured, by passing a unique label to each axes instance.
ax = fh.add_axes(
Looping through selected volumes in a 4D file¶
If we want to plot selected volumes in this 4D file, we can use index_img with the slice constructor to select the desired volumes.
Afterwards, we’ll use iter_img to loop through them following the same formula as before.
selected_volumes = image.index_img(rsn, slice(3, 5))
If you’re new to Python, one thing to note is that the slice constructor uses 0-based indexing. You can confirm this by matching these slices to the previous plot above.
for img in image.iter_img(selected_volumes):
plot_stat_map(img)
plotting.show is useful to force the display of figures when running outside IPython
show()
To recap, neuroimaging images (niimgs as we call them) come in different flavors:
3D images, containing only one brain volume
4D images, containing multiple brain volumes.
More details about the input formats in nilearn for 3D and 4D images is given in the documentation section: Inputing data: file names or image objects.
Functions accept either 3D or 4D images, and we need to use on the one
hand index_img
or iter_img
to break down 4D images into 3D images, and on the other hand
concat_imgs
to group a list of 3D images into a 4D
image.
Total running time of the script: (0 minutes 22.159 seconds)
Estimated memory usage: 398 MB