Note
This page is a reference documentation. It only explains the class signature, and not how to use it. Please refer to the user guide for the big picture.
nilearn.connectome.ConnectivityMeasure#
- class nilearn.connectome.ConnectivityMeasure(cov_estimator=LedoitWolf(store_precision=False), kind='covariance', vectorize=False, discard_diagonal=False, standardize=True)[source]#
A class that computes different kinds of functional connectivity matrices.
New in version 0.2.
- Parameters:
- cov_estimatorestimator object, default=LedoitWolf(store_precision=False)
The covariance estimator. This implies that correlations are slightly shrunk towards zero compared to a maximum-likelihood estimate
- kind{“covariance”, “correlation”, “partial correlation”, “tangent”, “precision”}, default=’covariance’
The matrix kind. For the use of “tangent” see Varoquaux et al.[1].
- vectorizebool, default=False
If True, connectivity matrices are reshaped into 1D arrays and only their flattened lower triangular parts are returned.
- discard_diagonalbool, default=False
If True, vectorized connectivity coefficients do not include the matrices diagonal elements. Used only when vectorize is set to True.
- standardize
bool
, default=True If standardize is True, the data are centered and normed: their mean is put to 0 and their variance is put to 1 in the time dimension.
Note
Added to control passing value to standardize of
signal.clean
to call new behavior since passing “zscore” or True (default) is deprecated. This parameter will be deprecated in version 0.13 and removed in version 0.15.
References
- Attributes:
- cov_estimator_estimator object
A new covariance estimator with the same parameters as cov_estimator.
- mean_numpy.ndarray
The mean connectivity matrix across subjects. For ‘tangent’ kind, it is the geometric mean of covariances (a group covariance matrix that captures information from both correlation and partial correlation matrices). For other values for “kind”, it is the mean of the corresponding matrices
- whitening_numpy.ndarray
The inverted square-rooted geometric mean of the covariance matrices.
- __init__(cov_estimator=LedoitWolf(store_precision=False), kind='covariance', vectorize=False, discard_diagonal=False, standardize=True)[source]#
- fit(X, y=None)[source]#
Fit the covariance estimator to the given time series for each subject.
- Parameters:
- Xlist of numpy.ndarray, shape for each (n_samples, n_features)
The input subjects time series. The number of samples may differ from one subject to another.
- Returns:
- selfConnectivityMatrix instance
The object itself. Useful for chaining operations.
- fit_transform(X, y=None, confounds=None)[source]#
Fit the covariance estimator to the given time series for each subject. Then apply transform to covariance matrices for the chosen kind.
- Parameters:
- Xlist of n_subjects numpy.ndarray with shapes (n_samples, n_features)
The input subjects time series. The number of samples may differ from one subject to another.
- confoundsnp.ndarray with shape (n_samples) or (n_samples, n_confounds), or pandas DataFrame, optional
Confounds to be cleaned on the vectorized matrices. Only takes into effect when vetorize=True. This parameter is passed to signal.clean. Please see the related documentation for details.
- Returns:
- outputnumpy.ndarray, shape (n_subjects, n_features, n_features) or (n_subjects, n_features * (n_features + 1) / 2) if vectorize is set to True.
The transformed individual connectivities, as matrices or vectors. Vectors are cleaned when vectorize=True and confounds are provided.
- transform(X, confounds=None)[source]#
Apply transform to covariances matrices to get the connectivity matrices for the chosen kind.
- Parameters:
- Xlist of n_subjects numpy.ndarray with shapes (n_samples, n_features)
The input subjects time series. The number of samples may differ from one subject to another.
- confoundsnumpy.ndarray with shape (n_samples) or (n_samples, n_confounds), optional
Confounds to be cleaned on the vectorized matrices. Only takes into effect when vetorize=True. This parameter is passed to signal.clean. Please see the related documentation for details.
- Returns:
- outputnumpy.ndarray, shape (n_subjects, n_features, n_features) or (n_subjects, n_features * (n_features + 1) / 2) if vectorize is set to True.
The transformed individual connectivities, as matrices or vectors. Vectors are cleaned when vectorize=True and confounds are provided.
- inverse_transform(connectivities, diagonal=None)[source]#
Return connectivity matrices from connectivities, vectorized or not.
If kind is ‘tangent’, the covariance matrices are reconstructed.
- Parameters:
- connectivitieslist of n_subjects numpy.ndarray with shapes (n_features, n_features) or (n_features * (n_features + 1) / 2,)
or ((n_features - 1) * n_features / 2,) Connectivities of each subject, vectorized or not.
- diagonalnumpy.ndarray, shape (n_subjects, n_features), optional
The diagonals of the connectivity matrices.
- Returns:
- outputnumpy.ndarray, shape (n_subjects, n_features, n_features)
The corresponding connectivity matrices. If kind is ‘correlation’/ ‘partial correlation’, the correlation/partial correlation matrices are returned. If kind is ‘tangent’, the covariance matrices are reconstructed.
- get_metadata_routing()#
Get metadata routing of this object.
Please check User Guide on how the routing mechanism works.
- Returns:
- routingMetadataRequest
A
MetadataRequest
encapsulating routing information.
- get_params(deep=True)#
Get parameters for this estimator.
- Parameters:
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns:
- paramsdict
Parameter names mapped to their values.
- set_inverse_transform_request(*, connectivities='$UNCHANGED$', diagonal='$UNCHANGED$')#
Request metadata passed to the
inverse_transform
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed toinverse_transform
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it toinverse_transform
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- connectivitiesstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
connectivities
parameter ininverse_transform
.- diagonalstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
diagonal
parameter ininverse_transform
.
- Returns:
- selfobject
The updated object.
- set_output(*, transform=None)#
Set output container.
See Introducing the set_output API for an example on how to use the API.
- Parameters:
- transform{“default”, “pandas”}, default=None
Configure output of transform and fit_transform.
“default”: Default output format of a transformer
“pandas”: DataFrame output
“polars”: Polars output
None: Transform configuration is unchanged
New in version 1.4: “polars” option was added.
- Returns:
- selfestimator instance
Estimator instance.
- set_params(**params)#
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters:
- **paramsdict
Estimator parameters.
- Returns:
- selfestimator instance
Estimator instance.
- set_transform_request(*, confounds='$UNCHANGED$')#
Request metadata passed to the
transform
method.Note that this method is only relevant if
enable_metadata_routing=True
(seesklearn.set_config
). Please see User Guide on how the routing mechanism works.The options for each parameter are:
True
: metadata is requested, and passed totransform
if provided. The request is ignored if metadata is not provided.False
: metadata is not requested and the meta-estimator will not pass it totransform
.None
: metadata is not requested, and the meta-estimator will raise an error if the user provides it.str
: metadata should be passed to the meta-estimator with this given alias instead of the original name.
The default (
sklearn.utils.metadata_routing.UNCHANGED
) retains the existing request. This allows you to change the request for some parameters and not others.New in version 1.3.
Note
This method is only relevant if this estimator is used as a sub-estimator of a meta-estimator, e.g. used inside a
Pipeline
. Otherwise it has no effect.- Parameters:
- confoundsstr, True, False, or None, default=sklearn.utils.metadata_routing.UNCHANGED
Metadata routing for
confounds
parameter intransform
.
- Returns:
- selfobject
The updated object.
Examples using nilearn.connectome.ConnectivityMeasure
#
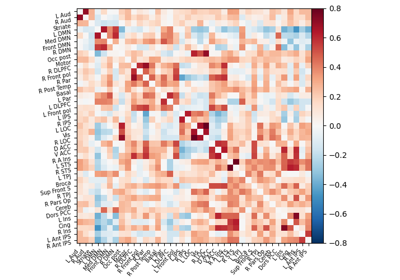
Extracting signals of a probabilistic atlas of functional regions
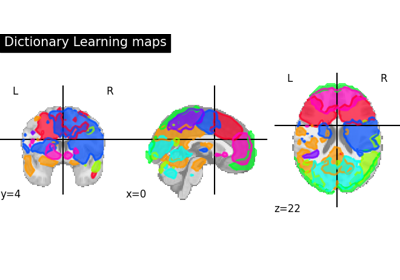
Regions extraction using dictionary learning and functional connectomes
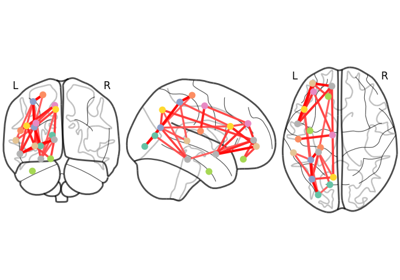
Comparing connectomes on different reference atlases
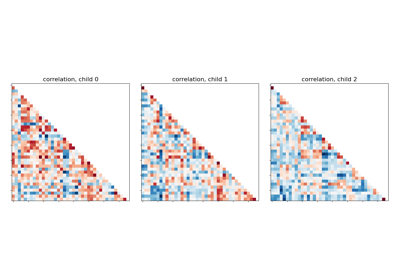
Classification of age groups using functional connectivity