Note
Go to the end to download the full example code. or to run this example in your browser via Binder
A introduction tutorial to fMRI decoding¶
Here is a simple tutorial on decoding with nilearn. It reproduces the Haxby et al.[1] study on a face vs cat discrimination task in a mask of the ventral stream.
This tutorial is meant as an introduction to the various steps of a decoding
analysis using Nilearn meta-estimator: Decoder
It is not a minimalistic example, as it strives to be didactic. It is not meant to be copied to analyze new data: many of the steps are unnecessary.
import warnings
warnings.filterwarnings(
"ignore", message="The provided image has no sform in its header."
)
Retrieve and load the fMRI data from the Haxby study¶
First download the data¶
The fetch_haxby
function will download the
Haxby dataset if not present on the disk, in the nilearn data directory.
It can take a while to download about 310 Mo of data from the Internet.
from nilearn import datasets
# By default 2nd subject will be fetched
haxby_dataset = datasets.fetch_haxby()
# 'func' is a list of filenames: one for each subject
fmri_filename = haxby_dataset.func[0]
# print basic information on the dataset
print(f"First subject functional nifti images (4D) are at: {fmri_filename}")
[fetch_haxby] Dataset created in /home/runner/nilearn_data/haxby2001
[fetch_haxby] Downloading data from
https://www.nitrc.org/frs/download.php/7868/mask.nii.gz ...
[fetch_haxby] ...done. (0 seconds, 0 min)
[fetch_haxby] Downloading data from
http://data.pymvpa.org/datasets/haxby2001/MD5SUMS ...
[fetch_haxby] ...done. (0 seconds, 0 min)
[fetch_haxby] Downloading data from
http://data.pymvpa.org/datasets/haxby2001/subj2-2010.01.14.tar.gz ...
[fetch_haxby] Downloaded 94961664 of 291168628 bytes (32.6%%, 2.1s remaining)
[fetch_haxby] Downloaded 214892544 of 291168628 bytes (73.8%%, 0.7s
remaining)
[fetch_haxby] ...done. (3 seconds, 0 min)
[fetch_haxby] Extracting data from
/home/runner/nilearn_data/haxby2001/9cabe068089e791ef0c5fe930fc20e30/subj2-2010.
01.14.tar.gz...
[fetch_haxby] .. done.
First subject functional nifti images (4D) are at: /home/runner/nilearn_data/haxby2001/subj2/bold.nii.gz
Visualizing the fMRI volume¶
One way to visualize a fMRI volume is
using plot_epi
.
We will visualize the previously fetched fMRI
data from Haxby dataset.
Because fMRI data are 4D
(they consist of many 3D EPI images),
we cannot plot them directly using plot_epi
(which accepts just 3D input).
Here we are using mean_img
to
extract a single 3D EPI image from the fMRI data.
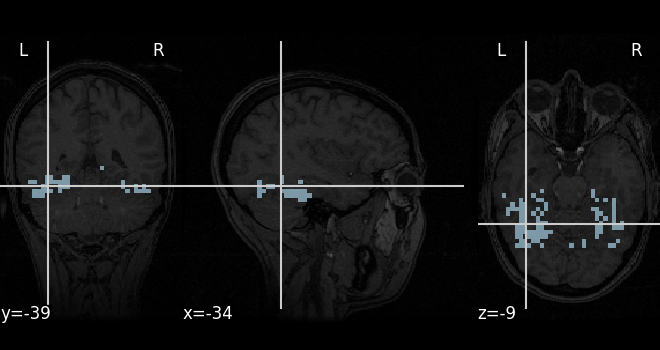
Feature extraction: from fMRI volumes to a data matrix¶
These are some really lovely images, but for machine learning
we need matrices to work with the actual data. Fortunately, the
Decoder
object we will use later on can
automatically transform Nifti images into matrices.
All we have to do for now is define a mask filename.
A mask of the Ventral Temporal (VT) cortex coming from the Haxby study is available:
mask_filename = haxby_dataset.mask_vt[0]
# Let's visualize it, using the subject's anatomical image as a
# background
plot_roi(mask_filename, bg_img=haxby_dataset.anat[0], cmap="Paired")
show()
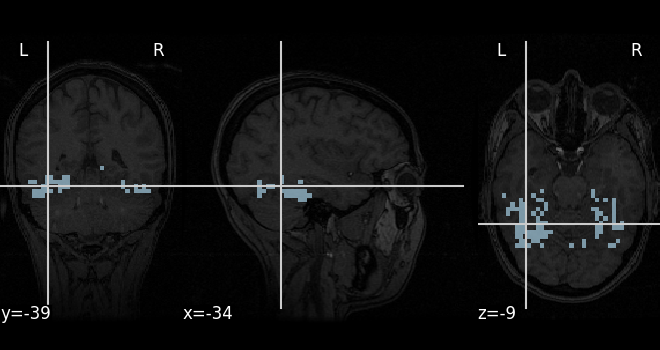
Load the behavioral labels¶
Now that the brain images are converted to a data matrix, we can apply machine-learning to them, for instance to predict the task that the subject was doing. The behavioral labels are stored in a CSV file, separated by spaces.
We use pandas to load them in an array.
import pandas as pd
# Load behavioral information
behavioral = pd.read_csv(haxby_dataset.session_target[0], delimiter=" ")
print(behavioral)
labels chunks
0 rest 0
1 rest 0
2 rest 0
3 rest 0
4 rest 0
... ... ...
1447 rest 11
1448 rest 11
1449 rest 11
1450 rest 11
1451 rest 11
[1452 rows x 2 columns]
The task was a visual-recognition task, and the labels denote the experimental condition: the type of object that was presented to the subject. This is what we are going to try to predict.
conditions = behavioral["labels"]
print(conditions)
0 rest
1 rest
2 rest
3 rest
4 rest
...
1447 rest
1448 rest
1449 rest
1450 rest
1451 rest
Name: labels, Length: 1452, dtype: object
Restrict the analysis to cats and faces¶
As we can see from the targets above, the experiment contains many conditions. As a consequence, the data is quite big. Not all of this data has an interest to us for decoding, so we will keep only fMRI signals corresponding to faces or cats. We create a mask of the samples belonging to the condition; this mask is then applied to the fMRI data to restrict the classification to the face vs cat discrimination.
The input data will become much smaller (i.e. fMRI signal is shorter):
condition_mask = conditions.isin(["face", "cat"])
Because the data is in one single large 4D image, we need to use index_img to do the split easily.
from nilearn.image import index_img
fmri_niimgs = index_img(fmri_filename, condition_mask)
We apply the same mask to the targets
conditions = conditions[condition_mask]
conditions = conditions.to_numpy()
print(f"{conditions.shape=}")
conditions.shape=(216,)
Decoding with Support Vector Machine¶
As a decoder, we use a Support Vector Classifier with a linear kernel. We
first create it using by using Decoder
.
from nilearn.decoding import Decoder
decoder = Decoder(
estimator="svc", mask=mask_filename, standardize="zscore_sample"
)
The decoder object is an object that can be fit (or trained) on data with labels, and then predict labels on data without.
We first fit it on the data
We can then predict the labels from the data
prediction = decoder.predict(fmri_niimgs)
print(f"{prediction=}")
prediction=array(['face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'cat', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'face', 'face', 'face', 'face', 'face',
'face', 'face', 'face', 'cat', 'cat', 'cat', 'cat', 'cat', 'cat',
'cat', 'cat', 'cat'], dtype='<U4')
Note that for this classification task both classes contain the same number of samples (the problem is balanced). Then, we can use accuracy to measure the performance of the decoder. This is done by defining accuracy as the scoring. Let’s measure the prediction accuracy:
print((prediction == conditions).sum() / float(len(conditions)))
1.0
This prediction accuracy score is meaningless. Why?
Measuring prediction scores using cross-validation¶
The proper way to measure error rates or prediction accuracy is via cross-validation: leaving out some data and testing on it.
Manually leaving out data¶
Let’s leave out the 30 last data points during training, and test the prediction on these 30 last points:
fmri_niimgs_train = index_img(fmri_niimgs, slice(0, -30))
fmri_niimgs_test = index_img(fmri_niimgs, slice(-30, None))
conditions_train = conditions[:-30]
conditions_test = conditions[-30:]
decoder = Decoder(
estimator="svc", mask=mask_filename, standardize="zscore_sample"
)
decoder.fit(fmri_niimgs_train, conditions_train)
prediction = decoder.predict(fmri_niimgs_test)
# The prediction accuracy is calculated on the test data: this is the accuracy
# of our model on examples it hasn't seen to examine how well the model perform
# in general.
predicton_accuracy = (prediction == conditions_test).sum() / float(
len(conditions_test)
)
print(f"Prediction Accuracy: {predicton_accuracy:.3f}")
Prediction Accuracy: 0.767
Implementing a KFold loop¶
We can manually split the data in train and test set repetitively in a KFold strategy by importing scikit-learn’s object:
from sklearn.model_selection import KFold
cv = KFold(n_splits=5)
for fold, (train, test) in enumerate(cv.split(conditions), start=1):
decoder = Decoder(
estimator="svc", mask=mask_filename, standardize="zscore_sample"
)
decoder.fit(index_img(fmri_niimgs, train), conditions[train])
prediction = decoder.predict(index_img(fmri_niimgs, test))
predicton_accuracy = (prediction == conditions[test]).sum() / float(
len(conditions[test])
)
print(
f"CV Fold {fold:01d} | Prediction Accuracy: {predicton_accuracy:.3f}"
)
CV Fold 1 | Prediction Accuracy: 0.886
CV Fold 2 | Prediction Accuracy: 0.767
CV Fold 3 | Prediction Accuracy: 0.767
CV Fold 4 | Prediction Accuracy: 0.698
CV Fold 5 | Prediction Accuracy: 0.744
Cross-validation with the decoder¶
The decoder also implements a cross-validation loop by default and returns an array of shape (cross-validation parameters, n_folds). We can use accuracy score to measure its performance by defining accuracy as the scoring parameter.
n_folds = 5
decoder = Decoder(
estimator="svc",
mask=mask_filename,
standardize="zscore_sample",
cv=n_folds,
scoring="accuracy",
)
decoder.fit(fmri_niimgs, conditions)
Cross-validation pipeline can also be implemented manually. More details can be found on scikit-learn website.
Then we can check the best performing parameters per fold.
print(decoder.cv_params_["face"])
{'C': [100.0, 100.0, 100.0, 100.0, 100.0]}
Note
We can speed things up to use all the CPUs of our computer with the n_jobs parameter.
The best way to do cross-validation is to respect the structure of the experiment, for instance by leaving out full runs of acquisition.
The number of the run is stored in the CSV file giving the behavioral data. We have to apply our run mask, to select only cats and faces.
run_label = behavioral["chunks"][condition_mask]
The fMRI data is acquired by runs, and the noise is autocorrelated in a given run. Hence, it is better to predict across runs when doing cross-validation. To leave a run out, pass the cross-validator object to the cv parameter of decoder.
from sklearn.model_selection import LeaveOneGroupOut
cv = LeaveOneGroupOut()
decoder = Decoder(
estimator="svc", mask=mask_filename, standardize="zscore_sample", cv=cv
)
decoder.fit(fmri_niimgs, conditions, groups=run_label)
print(f"{decoder.cv_scores_=}")
decoder.cv_scores_={'cat': [1.0, 1.0, 1.0, 1.0, 0.9629629629629629, 0.8518518518518519, 0.9753086419753086, 0.40740740740740744, 0.9876543209876543, 1.0, 0.9259259259259259, 0.8765432098765432], 'face': [1.0, 1.0, 1.0, 1.0, 0.9629629629629629, 0.8518518518518519, 0.9753086419753086, 0.40740740740740744, 0.9876543209876543, 1.0, 0.9259259259259259, 0.8765432098765432]}
Inspecting the model weights¶
Finally, it may be useful to inspect and display the model weights.
Turning the weights into a nifti image¶
We retrieve the SVC discriminating weights
coef_ = decoder.coef_
print(f"{coef_=}")
coef_=array([[-3.89375126e-02, -1.87167218e-02, -3.23027615e-02,
-2.88745540e-02, 4.18695944e-02, 1.10745058e-02,
1.69997889e-02, -5.50955637e-02, -1.94205305e-02,
-3.51225226e-02, 1.08512535e-02, -1.28797726e-02,
-1.54676965e-02, -3.78907409e-02, -3.69169634e-02,
2.28088054e-02, 6.56427052e-03, -7.65752905e-03,
1.67105969e-02, -8.02157239e-03, 5.29514245e-02,
-8.17596249e-02, -6.36992713e-02, 2.41324970e-02,
4.59874120e-02, -2.22602911e-02, -1.77308903e-02,
2.22195616e-02, -9.53208745e-03, 5.76044812e-02,
2.14299579e-02, -9.14226218e-02, 4.03666383e-03,
-2.89274219e-02, -3.89028154e-02, -3.35115343e-02,
2.21397400e-03, 8.73139039e-03, -3.37414248e-02,
-2.41270943e-02, -6.81647364e-02, 1.65407923e-02,
2.70784182e-02, -6.56857495e-03, -1.21662597e-02,
5.47676140e-02, 8.13257264e-03, 3.60957170e-02,
-1.52762064e-02, 7.02913211e-02, 1.28114611e-03,
2.08008668e-02, -4.09947092e-03, 3.72430651e-02,
-3.77395268e-02, -1.03857664e-02, -2.38236741e-02,
-5.48881013e-02, 4.43027857e-02, -1.47419202e-01,
-2.34044518e-02, 1.87113567e-02, 6.65861951e-02,
-9.07601573e-02, -1.22035248e-02, -2.95631185e-03,
3.22091550e-02, -3.04054119e-02, 6.15347226e-02,
1.12246789e-02, 1.93776824e-02, -1.30542862e-02,
4.42975770e-02, -2.23065920e-02, 6.88147751e-02,
1.69390846e-02, 1.78947987e-02, 1.00277403e-02,
2.99186920e-02, -2.52171655e-02, 1.06154126e-02,
-6.31949764e-03, 2.21521149e-03, -2.23349002e-02,
1.42561489e-02, -1.53122520e-02, -1.98226593e-02,
-4.32639564e-02, -4.55124385e-02, 3.41590401e-02,
-2.79198925e-02, -2.80912506e-02, -3.70160575e-02,
-5.71450714e-02, -6.98952030e-02, 3.20157133e-03,
-8.35407552e-03, -3.37626117e-02, 3.04262088e-02,
8.68460315e-03, 6.19383995e-03, 5.94177422e-02,
9.07301415e-03, -1.48932287e-02, 1.43559941e-02,
-1.09027601e-02, 2.67699153e-02, 4.73789183e-02,
-2.96432915e-02, 3.09421447e-02, 1.57926873e-02,
-3.16718252e-02, -4.00105695e-02, -5.40261230e-02,
2.82612006e-02, -1.12099566e-02, -5.45401490e-02,
6.32177451e-02, -1.49997425e-02, 2.47551020e-03,
-4.56646108e-02, -1.83882239e-02, 1.19956730e-02,
-3.72173190e-02, -2.25528157e-03, 4.58656166e-02,
4.79164353e-02, 2.51817256e-03, -4.31720677e-02,
-5.35328510e-03, 5.76993080e-02, 7.40838049e-03,
-3.20591381e-02, 4.35699482e-03, 1.68303282e-02,
-2.92570123e-02, -2.24487095e-03, -8.30214614e-03,
-1.00014013e-02, 2.17134676e-02, -1.92624802e-03,
-1.33222022e-02, -2.80297066e-02, -1.75291227e-02,
-9.17775904e-03, -7.09920691e-03, -1.43034646e-02,
5.06834063e-02, -1.84814175e-02, -4.71510539e-02,
1.72569691e-02, -4.76643026e-02, -9.09233710e-04,
4.00771288e-02, 7.53993630e-02, 7.25606390e-03,
4.82605787e-02, 4.50556076e-02, 3.61203557e-02,
-8.16483345e-03, 1.95407454e-02, 3.57883261e-02,
4.89306945e-02, 3.82972814e-02, 6.23921219e-02,
6.13674695e-02, -1.68752130e-02, 1.66515230e-02,
3.35525125e-02, -1.80213356e-02, 4.46410407e-02,
-3.53244983e-02, -3.67291621e-02, -4.62264479e-03,
4.86833778e-02, 3.39667561e-02, 6.21707928e-03,
1.73611685e-02, 2.01697061e-02, 2.17096754e-02,
2.91414648e-02, 2.37776881e-02, 4.84698414e-02,
-9.22625320e-03, -2.82637005e-02, -2.13781038e-02,
1.80777147e-03, 4.79691063e-02, -9.78906680e-03,
1.11430613e-02, -1.65021658e-02, -2.89088452e-02,
2.42849455e-02, -1.22346065e-02, -2.92869369e-02,
-2.89845826e-02, -3.39531517e-02, -3.65298970e-03,
2.65321223e-02, 4.58040193e-02, -5.93381923e-02,
-2.13629029e-02, -3.09403716e-02, 5.50176807e-02,
-3.38816802e-02, 6.12629963e-03, 1.41485402e-02,
1.10217703e-02, 5.33810524e-02, -2.12339140e-02,
6.37414810e-03, -1.13076037e-02, -2.64225572e-02,
-2.22398294e-02, -5.31920301e-02, -3.98652248e-02,
-1.29727641e-01, -3.28091026e-02, -2.89710544e-02,
-9.13466644e-03, -7.28724185e-03, -3.71051417e-02,
-6.34909434e-02, 2.04400154e-03, -8.26794821e-02,
-6.71216422e-02, -2.29119144e-03, -2.33450834e-02,
1.77914749e-02, -8.74665909e-02, -2.76483997e-03,
-4.38275847e-02, -1.28050611e-02, 2.78032588e-02,
-4.32695653e-02, -3.22687464e-02, -2.28027951e-02,
-2.57412817e-02, 2.03623153e-02, -9.90250392e-03,
-3.15035380e-02, -1.81420123e-02, -1.12300284e-03,
-4.17432596e-02, -6.23478951e-02, 2.54602997e-04,
-6.73679728e-02, 6.53966004e-02, 1.06523616e-02,
2.21983192e-02, -1.98727396e-02, -1.85517124e-02,
4.05701303e-02, -3.02835823e-02, -8.10051913e-02,
-7.42456892e-02, -4.93849845e-02, -1.01770505e-02,
1.09409866e-02, -4.49254234e-02, 2.92749200e-02,
7.05314520e-03, 5.07552619e-03, -4.84040848e-03,
2.48721329e-03, 3.00654970e-02, -2.63093563e-03,
4.64685305e-03, 7.90210242e-02, 1.04856477e-02,
1.68079754e-02, -4.36718181e-02, -1.08855765e-02,
2.10238171e-02, -4.41964136e-02, 3.16491215e-03,
6.98670784e-02, 8.61632632e-02, 4.96231777e-02,
6.03886858e-03, 5.56494400e-02, -2.98920434e-02,
4.13033930e-03, -3.21953501e-02, -3.14991367e-02,
-5.31277598e-02, 2.67256143e-02, 3.14427544e-02,
6.67122360e-03, -1.28702933e-02, 2.20150635e-02,
5.68522183e-02, 2.25602832e-02, -2.04617569e-02,
5.10330705e-03, 2.85358084e-02, -1.81664867e-02,
-8.48451892e-03, -3.18824589e-02, -1.18499550e-02,
-4.10843998e-02, 3.11780921e-02, 9.63460270e-03,
-8.25914064e-03, -3.12226877e-02, 8.57659657e-03,
-9.70196567e-03, 1.32373073e-02, 4.06446101e-02,
8.23369212e-03, -3.27356840e-02, -4.33862142e-03,
-1.75529515e-02, 6.88839546e-03, 3.45132246e-02,
7.03297904e-02, 2.16787461e-02, 5.32224254e-03,
8.17561803e-02, 6.40062816e-02, -2.31152587e-03,
-1.17557180e-02, 1.75888996e-01, 3.18128742e-02,
-3.15887292e-02, 3.34028570e-02, 2.22783180e-02,
1.00231733e-02, -4.74911888e-02, -2.12759529e-02,
-3.98716995e-02, -6.04067051e-02, -4.65061109e-02,
1.03001752e-02, -3.05481512e-04, 1.80743321e-02,
-1.75451581e-02, -8.72589832e-02, 1.00662531e-01,
4.46113136e-03, 7.46871881e-02, -6.13409540e-02,
2.81707103e-02, -1.40976207e-02, 3.14637643e-02,
-1.63833277e-02, 3.66532027e-02, -5.15682974e-03,
1.45093561e-02, 6.35863612e-02, 2.34599331e-02,
8.81063365e-02, 6.15345362e-02, -1.39360315e-02,
2.07247557e-02, -3.15472673e-03, 5.15424973e-02,
-2.88767858e-02, 1.60263655e-02, 2.09701913e-02,
-3.29173438e-02, -2.59462318e-02, -5.60400065e-02,
-3.64629389e-02, 1.12881803e-02, 2.17267024e-02,
-1.51636529e-02, -7.82900272e-03, 2.42550409e-02,
9.47013651e-02, -2.63032597e-02, 1.17260358e-04,
-5.24185743e-03, 4.17986854e-02, 8.85679631e-02,
6.23656020e-03, 1.86601126e-02, 1.54628971e-02,
3.50530243e-03, 6.20586724e-03, -1.19788926e-02,
1.59526150e-02, 7.12140974e-03, -8.93192799e-02,
-3.54347717e-03, 1.23477646e-02, 3.03927259e-02,
-2.37292035e-02, -3.82794712e-02, -4.98745039e-02,
4.66898150e-02, -1.23293465e-02, -1.10332355e-02,
2.18106616e-02, 2.18722552e-02, 2.63537981e-02,
1.05280978e-02, 1.84617986e-02, 8.36058902e-04,
-6.65206530e-03, 3.49396995e-02, 1.49353189e-02,
-1.11600024e-02, 6.69119020e-03, -2.00058404e-02,
-3.99014163e-02, 3.01872879e-02, -1.09867765e-02,
-4.11779912e-02, 2.72052238e-02, 1.16425507e-02,
-1.55501598e-02, 3.27700599e-02, 3.95494138e-02,
8.48736864e-03, 2.19937068e-02, -9.88684040e-03,
-3.61421494e-02, -4.77020992e-02, 1.90071462e-02,
-5.58287340e-02, -3.31736934e-02, -2.24915382e-02,
-3.36175178e-02, -4.07356964e-02, 1.08861216e-02,
1.12812578e-02, 7.63144255e-02, 4.04811635e-03,
3.07012916e-02, 2.89178762e-02, 4.71595190e-03,
5.13384191e-02, -4.10364156e-02, 1.23264715e-03,
-2.50402072e-02, 5.85904187e-02, -1.04965689e-01,
-4.41706299e-02, 1.18518706e-02, -5.83202290e-02,
-4.82244718e-02, 9.17680863e-03, 1.03260861e-02,
-5.09174617e-03, -3.23390693e-02, -3.19382987e-02,
-1.53771708e-02, -5.21213869e-02, 1.55620163e-02,
2.93484990e-02, -1.92527395e-02, 1.76696994e-02,
2.67992755e-02, 5.76552554e-02, -1.38162078e-02,
2.60398748e-02, 1.50400963e-02, 1.27424073e-02,
-2.29243999e-02, -1.06666863e-02, 9.81927620e-03,
-4.77513212e-02, 1.64241783e-02]])
It’s a numpy array with only one coefficient per voxel:
print(f"{coef_.shape=}")
coef_.shape=(1, 464)
To get the Nifti image of these coefficients, we only need retrieve the coef_img_ in the decoder and select the class
coef_img = decoder.coef_img_["face"]
coef_img is now a NiftiImage. We can save the coefficients as a nii.gz file:
from pathlib import Path
output_dir = Path.cwd() / "results" / "plot_decoding_tutorial"
output_dir.mkdir(exist_ok=True, parents=True)
print(f"Output will be saved to: {output_dir}")
decoder.coef_img_["face"].to_filename(output_dir / "haxby_svc_weights.nii.gz")
Output will be saved to: /home/runner/work/nilearn/nilearn/examples/00_tutorials/results/plot_decoding_tutorial
Plotting the SVM weights¶
We can plot the weights, using the subject’s anatomical as a background
from nilearn.plotting import view_img
view_img(
decoder.coef_img_["face"],
bg_img=haxby_dataset.anat[0],
title="SVM weights",
dim=-1,
)
/home/runner/work/nilearn/nilearn/.tox/doc/lib/python3.9/site-packages/numpy/core/fromnumeric.py:758: UserWarning: Warning: 'partition' will ignore the 'mask' of the MaskedArray.
a.partition(kth, axis=axis, kind=kind, order=order)