Note
Click here to download the full example code or to run this example in your browser via Binder
9.4.6. Comparing connectomes on different reference atlases¶
This examples shows how to turn a parcellation into connectome for visualization. This requires choosing centers for each parcel or network, via nilearn.plotting.find_parcellation_cut_coords
for parcellation based on labels and nilearn.plotting.find_probabilistic_atlas_cut_coords
for parcellation based on probabilistic values.
In the intermediary steps, we make use of nilearn.input_data.NiftiLabelsMasker
and nilearn.input_data.NiftiMapsMasker
to extract time series from nifti objects using different parcellation atlases.
The time series of all subjects of the brain development dataset are concatenated and given directly to nilearn.connectome.ConnectivityMeasure
for computing parcel-wise correlation matrices for each atlas across all subjects.
Mean correlation matrix is displayed on glass brain on extracted coordinates.
# author: Amadeus Kanaan
9.4.6.1. Load atlases¶
from nilearn import datasets
yeo = datasets.fetch_atlas_yeo_2011()
print('Yeo atlas nifti image (3D) with 17 parcels and liberal mask is located '
'at: %s' % yeo['thick_17'])
Out:
Dataset created in /home/varoquau/nilearn_data/yeo_2011
Downloading data from ftp://surfer.nmr.mgh.harvard.edu/pub/data/Yeo_JNeurophysiol11_MNI152.zip ...
Downloaded 802816 of ? bytes. ...done. (3 seconds, 0 min)
Extracting data from /home/varoquau/nilearn_data/yeo_2011/d7a5390bfb7686fb41fa64cc2ba058d0/Yeo_JNeurophysiol11_MNI152.zip..... done.
Yeo atlas nifti image (3D) with 17 parcels and liberal mask is located at: /home/varoquau/nilearn_data/yeo_2011/Yeo_JNeurophysiol11_MNI152/Yeo2011_17Networks_MNI152_FreeSurferConformed1mm_LiberalMask.nii.gz
9.4.6.2. Load functional data¶
data = datasets.fetch_development_fmri(n_subjects=10)
print('Functional nifti images (4D, e.g., one subject) are located at : %r'
% data['func'][0])
print('Counfound csv files (of same subject) are located at : %r'
% data['confounds'][0])
Out:
Functional nifti images (4D, e.g., one subject) are located at : '/home/varoquau/nilearn_data/development_fmri/development_fmri/sub-pixar123_task-pixar_space-MNI152NLin2009cAsym_desc-preproc_bold.nii.gz'
Counfound csv files (of same subject) are located at : '/home/varoquau/nilearn_data/development_fmri/development_fmri/sub-pixar123_task-pixar_desc-reducedConfounds_regressors.tsv'
9.4.6.3. Extract coordinates on Yeo atlas - parcellations¶
from nilearn.input_data import NiftiLabelsMasker
from nilearn.connectome import ConnectivityMeasure
# ConenctivityMeasure from Nilearn uses simple 'correlation' to compute
# connectivity matrices for all subjects in a list
connectome_measure = ConnectivityMeasure(kind='correlation')
# useful for plotting connectivity interactions on glass brain
from nilearn import plotting
# create masker to extract functional data within atlas parcels
masker = NiftiLabelsMasker(labels_img=yeo['thick_17'], standardize=True,
memory='nilearn_cache')
# extract time series from all subjects and concatenate them
time_series = []
for func, confounds in zip(data.func, data.confounds):
time_series.append(masker.fit_transform(func, confounds=confounds))
# calculate correlation matrices across subjects and display
correlation_matrices = connectome_measure.fit_transform(time_series)
# Mean correlation matrix across 10 subjects can be grabbed like this,
# using connectome measure object
mean_correlation_matrix = connectome_measure.mean_
# grab center coordinates for atlas labels
coordinates = plotting.find_parcellation_cut_coords(labels_img=yeo['thick_17'])
# plot connectome with 80% edge strength in the connectivity
plotting.plot_connectome(mean_correlation_matrix, coordinates,
edge_threshold="80%",
title='Yeo Atlas 17 thick (func)')
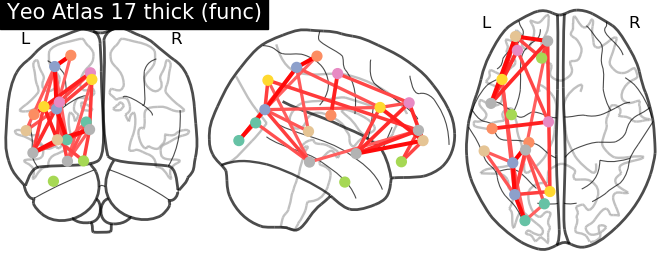
Out:
<nilearn.plotting.displays.OrthoProjector object at 0x7f8bfb3fcb80>
9.4.6.4. Load probabilistic atlases - extracting coordinates on brain maps¶
Out:
/usr/lib/python3/dist-packages/numpy/lib/npyio.py:2358: VisibleDeprecationWarning: Reading unicode strings without specifying the encoding argument is deprecated. Set the encoding, use None for the system default.
output = genfromtxt(fname, **kwargs)
9.4.6.5. Iterate over fetched atlases to extract coordinates - probabilistic¶
from nilearn.input_data import NiftiMapsMasker
# create masker to extract functional data within atlas parcels
masker = NiftiMapsMasker(maps_img=msdl['maps'], standardize=True,
memory='nilearn_cache')
# extract time series from all subjects and concatenate them
time_series = []
for func, confounds in zip(data.func, data.confounds):
time_series.append(masker.fit_transform(func, confounds=confounds))
# calculate correlation matrices across subjects and display
correlation_matrices = connectome_measure.fit_transform(time_series)
# Mean correlation matrix across 10 subjects can be grabbed like this,
# using connectome measure object
mean_correlation_matrix = connectome_measure.mean_
# grab center coordinates for probabilistic atlas
coordinates = plotting.find_probabilistic_atlas_cut_coords(maps_img=msdl['maps'])
# plot connectome with 80% edge strength in the connectivity
plotting.plot_connectome(mean_correlation_matrix, coordinates,
edge_threshold="80%", title='MSDL (probabilistic)')
plotting.show()
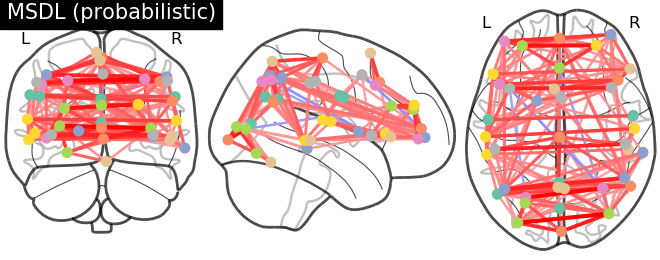
Out:
/home/varoquau/dev/nilearn/nilearn/_utils/cache_mixin.py:295: UserWarning: memory_level is currently set to 0 but a Memory object has been provided. Setting memory_level to 1.
warnings.warn("memory_level is currently set to 0 but "
Total running time of the script: ( 1 minutes 24.161 seconds)