Note
This page is a reference documentation. It only explains the class signature, and not how to use it. Please refer to the user guide for the big picture.
nilearn.maskers.MultiNiftiLabelsMasker#
- class nilearn.maskers.MultiNiftiLabelsMasker(labels_img, labels=None, background_label=0, mask_img=None, smoothing_fwhm=None, standardize=False, standardize_confounds=True, high_variance_confounds=False, detrend=False, low_pass=None, high_pass=None, t_r=None, dtype=None, resampling_target='data', memory=Memory(location=None), memory_level=1, verbose=0, strategy='mean', reports=True, n_jobs=1)[source]#
Class for masking of Niimg-like objects. MultiNiftiLabelsMasker is useful when data from non-overlapping volumes and from different subjects should be extracted (contrary to
nilearn.maskers.NiftiLabelsMasker
).- Parameters
- labels_imgNiimg-like object
See Input and output: neuroimaging data representation. Region definitions, as one image of labels.
- labels
list
ofstr
, optional Full labels corresponding to the labels image. This is used to improve reporting quality if provided.
Warning
The labels must be consistent with the label values provided through labels_img.
- background_label
int
orfloat
, optional Label used in labels_img to represent background. Warning: This value must be consistent with label values and image provided. Default=0.
- mask_imgNiimg-like object, optional
See Input and output: neuroimaging data representation. Mask to apply to regions before extracting signals.
- smoothing_fwhm
float
, optional. If
smoothing_fwhm
is notNone
, it gives the full-width at half maximum in millimeters of the spatial smoothing to apply to the signal.- standardize{‘zscore’, ‘psc’, True, False}, optional
Strategy to standardize the signal.
‘zscore’: the signal is z-scored. Timeseries are shifted to zero mean and scaled to unit variance.
‘psc’: Timeseries are shifted to zero mean value and scaled to percent signal change (as compared to original mean signal).
True : the signal is z-scored. Timeseries are shifted to zero mean and scaled to unit variance.
False : Do not standardize the data.
Default=False.
- standardize_confounds
bool
, optional If set to True, the confounds are z-scored: their mean is put to 0 and their variance to 1 in the time dimension. Default=True.
- high_variance_confounds
bool
, optional If True, high variance confounds are computed on provided image with
nilearn.image.high_variance_confounds
and default parameters and regressed out. Default=False.- detrend
bool
, optional Whether to detrend signals or not.
- low_pass
float
or None, optional Low cutoff frequency in Hertz. If specified, signals above this frequency will be filtered out. If None, no low-pass filtering will be performed. Default=None.
- high_pass
float
, optional High cutoff frequency in Hertz. If specified, signals below this frequency will be filtered out. Default=None.
- t_r
float
or None, optional Repetition time, in seconds (sampling period). Set to
None
if not provided. Default=None.- dtype{dtype, “auto”}
Data type toward which the data should be converted. If “auto”, the data will be converted to int32 if dtype is discrete and float32 if it is continuous.
- resampling_target{“data”, “labels”, None}, optional.
Gives which image gives the final shape/size:
“data” means the atlas is resampled to the shape of the data if needed
“labels” means en mask_img and images provided to fit() are resampled to the shape and affine of maps_img
None means no resampling: if shapes and affines do not match, a ValueError is raised
Default=”data”.
- memoryinstance of
joblib.Memory
,str
, orpathlib.Path
Used to cache the masking process. By default, no caching is done. If a
str
is given, it is the path to the caching directory.- memory_level
int
, optional. Rough estimator of the amount of memory used by caching. Higher value means more memory for caching. Zero means no caching. Default=0.
- n_jobs
int
, optional. The number of CPUs to use to do the computation. -1 means ‘all CPUs’. Default=1.
- verbose
int
, optional Verbosity level (0 means no message). Default=0.
- strategy
str
, optional The name of a valid function to reduce the region with. Must be one of: sum, mean, median, minimum, maximum, variance, standard_deviation. Default=’mean’.
- reports
bool
, optional If set to True, data is saved in order to produce a report. Default=True.
- __init__(labels_img, labels=None, background_label=0, mask_img=None, smoothing_fwhm=None, standardize=False, standardize_confounds=True, high_variance_confounds=False, detrend=False, low_pass=None, high_pass=None, t_r=None, dtype=None, resampling_target='data', memory=Memory(location=None), memory_level=1, verbose=0, strategy='mean', reports=True, n_jobs=1)[source]#
- transform_imgs(imgs_list, confounds=None, n_jobs=1, sample_mask=None)[source]#
Extract signals from a list of 4D niimgs.
- Parameters
- %(imgs)s
Images to process. Each element of the list is a 4D image.
- %(confounds)s
- %(sample_mask)s
- Returns
- region_signals: list of 2Dobj:numpy.ndarray
List of signals for each label per subject. shape: list of (number of scans, number of labels)
- transform(imgs, confounds=None, sample_mask=None)[source]#
Apply mask, spatial and temporal preprocessing
- Parameters
- %(imgs)s
Images to process. Each element of the list is a 4D image.
- %(confounds)s
- %(sample_mask)s
- Returns
- region_signalslist of 2D
numpy.ndarray
List of signals for each label per subject. shape: list of (number of scans, number of labels)
- region_signalslist of 2D
- fit(imgs=None, y=None)[source]#
Prepare signal extraction from regions.
All parameters are unused, they are for scikit-learn compatibility.
- fit_transform(imgs, confounds=None, sample_mask=None)[source]#
Prepare and perform signal extraction from regions.
- Parameters
- imgs3D/4D Niimg-like object
See Input and output: neuroimaging data representation. Images to process. If a 3D niimg is provided, a singleton dimension will be added to the output to represent the single scan in the niimg.
- confoundsCSV file or array-like or
pandas.DataFrame
, optional This parameter is passed to signal.clean. Please see the related documentation for details. shape: (number of scans, number of confounds)
- sample_maskAny type compatible with numpy-array indexing, optional
shape: (number of scans - number of volumes removed, ) Masks the niimgs along time/fourth dimension to perform scrubbing (remove volumes with high motion) and/or non-steady-state volumes. This parameter is passed to signal.clean.
New in version 0.8.0.
- Returns
- region_signals2D
numpy.ndarray
Signal for each label. shape: (number of scans, number of labels)
- region_signals2D
- get_params(deep=True)#
Get parameters for this estimator.
- Parameters
- deepbool, default=True
If True, will return the parameters for this estimator and contained subobjects that are estimators.
- Returns
- paramsdict
Parameter names mapped to their values.
- inverse_transform(signals)[source]#
Compute voxel signals from region signals
Any mask given at initialization is taken into account.
Changed in version 0.9.2: This method now supports 1D arrays, which will produce 3D images.
- Parameters
- signals1D/2D
numpy.ndarray
Signal for each region. If a 1D array is provided, then the shape should be (number of elements,), and a 3D img will be returned. If a 2D array is provided, then the shape should be (number of scans, number of elements), and a 4D img will be returned.
- signals1D/2D
- Returns
- img
nibabel.nifti1.Nifti1Image
Signal for each voxel shape: (X, Y, Z, number of scans)
- img
- set_output(*, transform=None)#
Set output container.
See Introducing the set_output API for an example on how to use the API.
- Parameters
- transform{“default”, “pandas”}, default=None
Configure output of transform and fit_transform.
“default”: Default output format of a transformer
“pandas”: DataFrame output
None: Transform configuration is unchanged
- Returns
- selfestimator instance
Estimator instance.
- set_params(**params)#
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as
Pipeline
). The latter have parameters of the form<component>__<parameter>
so that it’s possible to update each component of a nested object.- Parameters
- **paramsdict
Estimator parameters.
- Returns
- selfestimator instance
Estimator instance.
- transform_single_imgs(imgs, confounds=None, sample_mask=None)[source]#
Extract signals from a single 4D niimg.
- Parameters
- imgs3D/4D Niimg-like object
See Input and output: neuroimaging data representation. Images to process. If a 3D niimg is provided, a singleton dimension will be added to the output to represent the single scan in the niimg.
- confoundsCSV file or array-like or
pandas.DataFrame
, optional This parameter is passed to signal.clean. Please see the related documentation for details. shape: (number of scans, number of confounds)
- sample_maskAny type compatible with numpy-array indexing, optional
shape: (number of scans - number of volumes removed, ) Masks the niimgs along time/fourth dimension to perform scrubbing (remove volumes with high motion) and/or non-steady-state volumes. This parameter is passed to signal.clean.
New in version 0.8.0.
- Returns
- region_signals2D numpy.ndarray
Signal for each label. shape: (number of scans, number of labels)
- Warns
- DeprecationWarning
If a 3D niimg input is provided, the current behavior (adding a singleton dimension to produce a 2D array) is deprecated. Starting in version 0.12, a 1D array will be returned for 3D inputs.
Examples using nilearn.maskers.MultiNiftiLabelsMasker
#
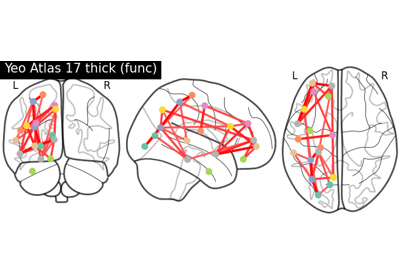
Comparing connectomes on different reference atlases